Objective: C program to perform Quick sort using the divide and conquer method
Concept: Quick Sort divides the array according to the value of elements. It rearranges elements of a given array A[0..n-1] to achieve its partition, where the elements before position s are smaller than or equal to A[s] and all the elements after position s are greater than or equal to A[s].
Algorithm:
Step 1: Start
Step 2: Declare the variables.
Step 3: Enter the elements to be sorted using the get()function.
Step 4: Divide the array list into two halves the lower array list and upper array list using the merge sort function.
Step 5: Sort the two array list.
Step 6: Combine the two sorted arrays.
Step 7: Display the sorted elements.
Step 8: Stop
Time complexities: Quick sort has an average time of O(n log n) on n elements. Its worst case time is O(n²)
#include
#include
void qsort();
int n;
void main()
{
int a[100],i,l,r;
clrscr();
printf("\nENTER THE SIZE OF THE ARRAY: ");
scanf("%d",&n);
for(i=0;ileft)
{
i=left;
j=right;
p=b[left];
finished=0;
while (!finished)
{
do
{
++i;
}
while ((b[i]<=p) && (i<=right));
while ((b[j]>=p) && (j>left))
{
--j;
}
if(j
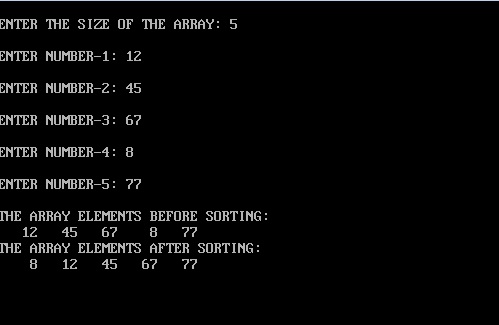