There are two methods for swapping:
- By using third variable.
- Without using third variable.
";
echo "a =".$a." b=".$b."
";
$third = $a;
$a = $b;
$b = $third;
echo "After swapping:
";
echo "a =".$a." b=".$b;
?>

Swapping Without using Third Variable
Swap two numbers without using a third variable is done in two ways:
- Using arithmetic operation + and
- Using arithmetic operation * and
- Using arithmetic operation + and –
";
echo "Value of a: $a";
echo "Value of b: $b";
$a=$a+$b;
$b=$a-$b;
$a=$a-$b;
echo " After Swapping:
";
echo "Value of a: $a";
echo "Value of b: $b";
?>
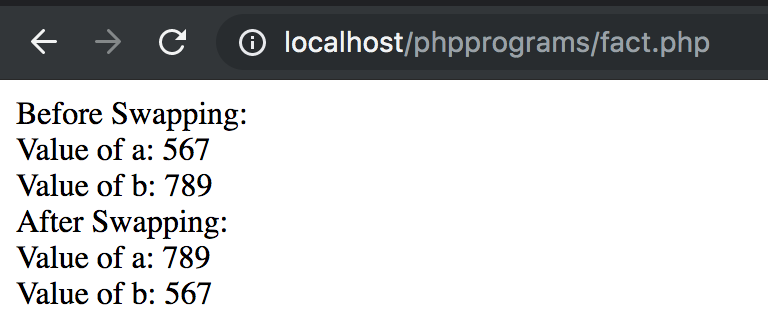
Using arithmetic operation * and /
";
echo "Value of a: $a";
echo "Value of b: $b";
$a=$a*$b;
$b=$a/$b;
$a=$a/$b;
echo " After Swapping:
";
echo "Value of a: $a";
echo "Value of b: $b"; ?>
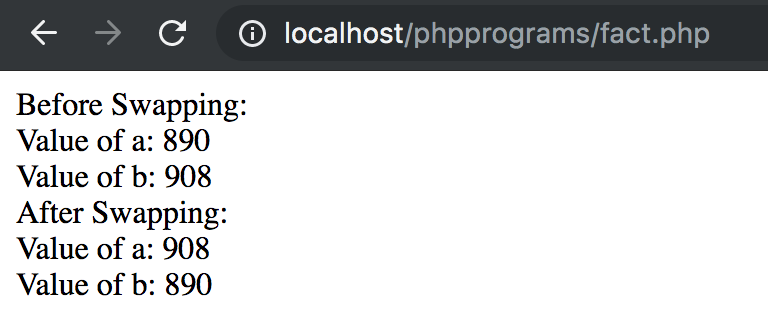